Trying to automate the Youtube live stream displaying in your website? Well… I had to deal with confusing GET requests to Youtube API with no success. Today I’m going to share the way to get the last video Id from a Youtube channel. It could help you to embed the livestreaming of your channel or show the last video published.
Youtube API
Sign up to your Google Cloud – Console account, create a new project and enable YouTube Data API v3. Additionally create and assign an API key to your project.
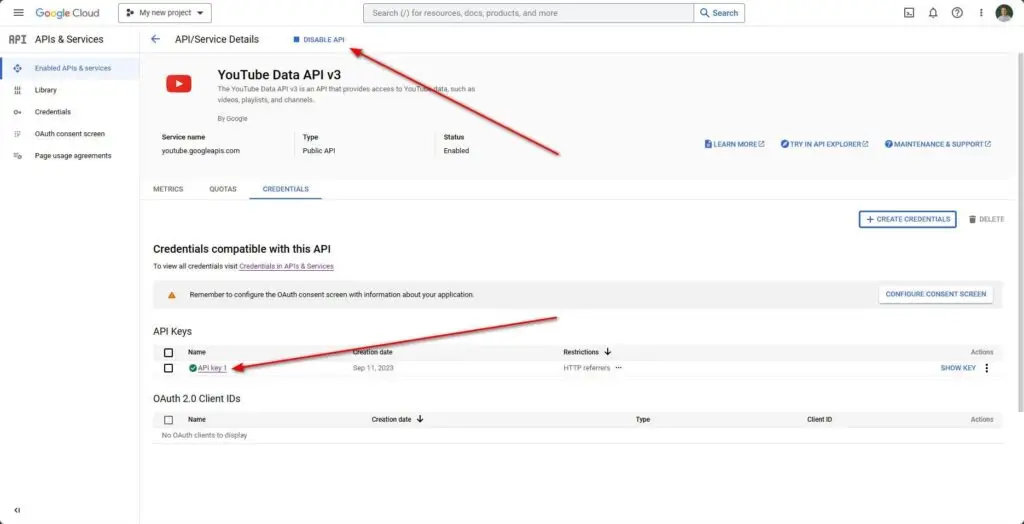
When you create a new API key for each project you should define an application restriction for that key. Application restriction lets you add the websites, IP addresses, android or iOS apps that are alowed to request data using your API key. If you left this option empty you are putting your quota usage at risk in case someone finds your API key and tries to use it.
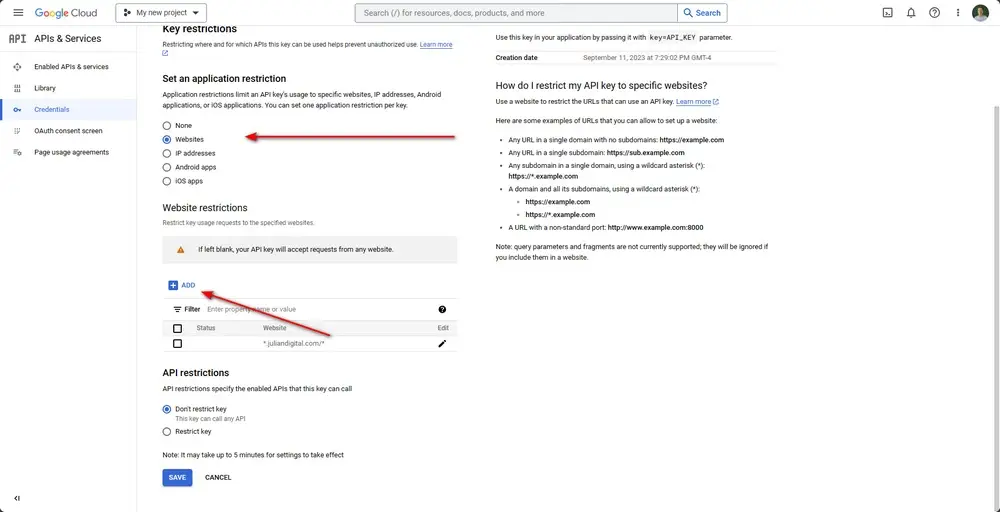
In case you are not sure of the traffic that could use the API and want to avoid extra fees edit the Quotas of the service.
Youtube channel Id
Find the channel id was tricky to me. I spend a lot of time requesting the API with the wrong cahnnel Id, finally I understood the username visible in your channel is not the same channel Id. Use YouTube Channel ID Finder to paste your @usernameChannel and find the channel Id.
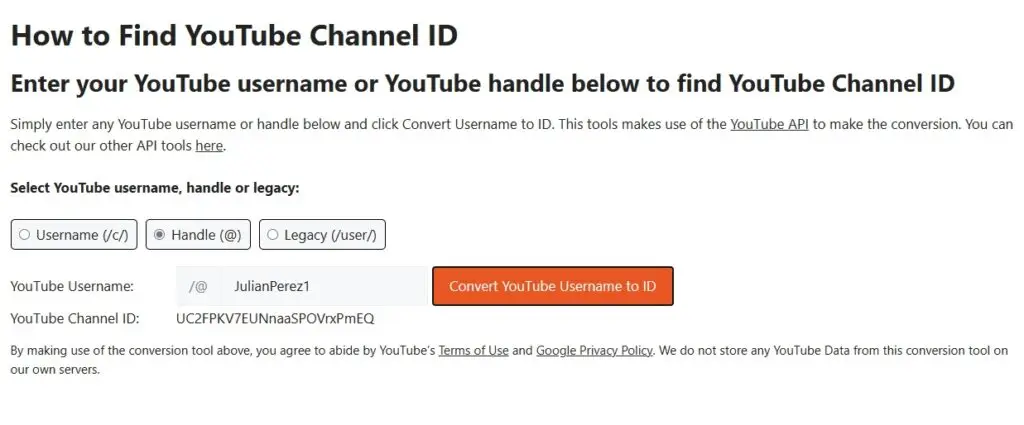
Javascript
Let’s create the javascript code:
<script>
function getYouTubeVideo() {
const apiKey = 'AIzaSyDsJgcnNQSFG5yAbCb0K6rgD4ZF-jhk'; // Replace with your YouTube API key
const channelId = 'UC2FPKV7EUNnaaSPOVrxPmEQ';
const maxResults = 1;
const currentTime = new Date().toLocaleString('en-US', { timeZone: 'America/Bogota' });
const currentDay = new Date(currentTime).getDay(); // 0 = Sunday, 1 = Monday, ..., 6 = Saturday
const currentHour = new Date(currentTime).getHours(); // Adjust for UTC-5 timezone
const iframeHeight = (window.innerWidth >= 768) ? '700px' : '350px';
// Check if it's Sunday after 12 PM (UTC-5)
if (currentDay === 7 && currentHour >= 13) {
const apiUrl = `https://www.googleapis.com/youtube/v3/search?channelId=${channelId}&order=date&maxResults=${maxResults}&key=${apiKey}`;
fetch(apiUrl)
.then(response => response.json())
.then(data => {
const videoId = data.items[0].id.videoId;
const embedCode = `
<iframe width="100%" height="${iframeHeight}" src="https://www.youtube.com/embed/${videoId}?&autoplay=1&mute=1" title="YouTube video player" frameborder="0" allow="accelerometer; autoplay; clipboard-write; encrypted-media; gyroscope; picture-in-picture; web-share" allowfullscreen></iframe>
`;
})
.catch(error => {
console.error('Error fetching YouTube data:', error);
});
}
}
// Call the function to load the video
getYouTubeVideo();
</script>
Notice I added one datetime validation because I needed to show the iframe only on Sundays at 1pm (Bogota time). Additionally I added the video height by parameter because I wanted to show the iframe smaller on mobile devices.
Hope It helps. Keep coding!