In the last months I was dealing with unexpected server overloads at any time of the week in a website running on an AWS Lightsail instance. The website receives high traffic on Sundays because there are events schedule every week that day. Sometimes the server was down on the morning, when the event was just starting or even during the event. I noticed the server was working slow hours or days before every crash. I suspected some plugin was malfunctioning adding a lot of work in the background, but I didn’t find relevant information after deactivating some of them. I suspected the site received DDoS attacks, but I am not definitely sure of that. The solution was always the same: reboot the instance, problem solved (at least for the moment).
After repeating this manual operation, I decided to schedule the rebooting everyday while I take the time to debug the website. Rebooting a Lightsail instance is a manual option enable in the UI, but I decided to program the operation with code.
I tried to look on the web for an easy tutorial but didn’t find a clearly step by step. After asking for help to GPT and reading a bit of documentation I found the solution and decide to share hoping it help someone.
Lambda
First of all, you require to create a new Lambda script where you are going to write the actions to do. Start opening Lambda service from the Console and click on Create function button.
We are going to create the function from scratch so name the script as you need, in my case rebootMyInsance, set the language to Python 3.10 or above, set the architecture to x86_64 and set execution role to Create a new role with basic Lambda permissions. Click on Create function button.
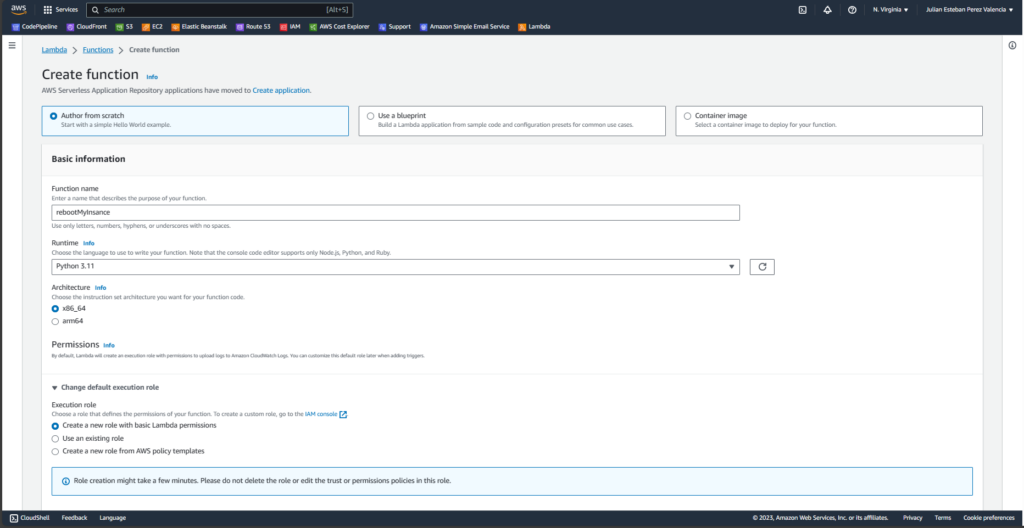
Inside the lambda_function tab we are going to paste the following script:
import boto3
def lambda_handler(event, context):
instance_name = "WordPress-JulianDigital"
client = boto3.client('lightsail')
response = client.reboot_instance(instanceName=instance_name)
return {
'statusCode': 200,
'body': 'Instance rebooted successfully.'
}
There are two relevant parts here: instance_name and reboot_instance.
- instance_name: is the literal name you defined for your Lightsail instance.
- reboot_instance: is the Boto3 method defined to reboot the instance. There is a lot of other ones to interact with your Lightsail instance.
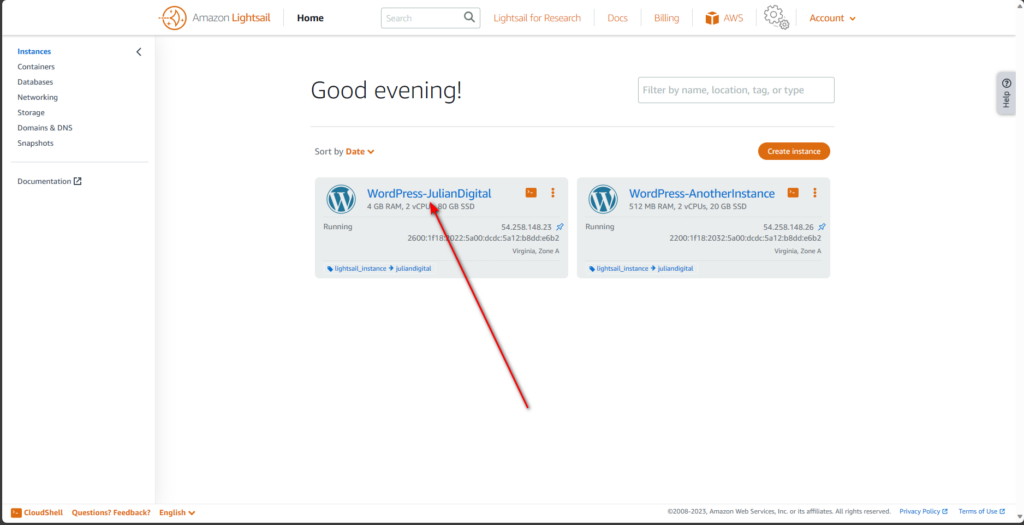
Every new Lambda function have its own role configuration. We need to set the appropriated role permissions to manipulate Lightsail. Go to Configuration tab and click on the Role name link. A new AWS console tab is opened with the role configuration.
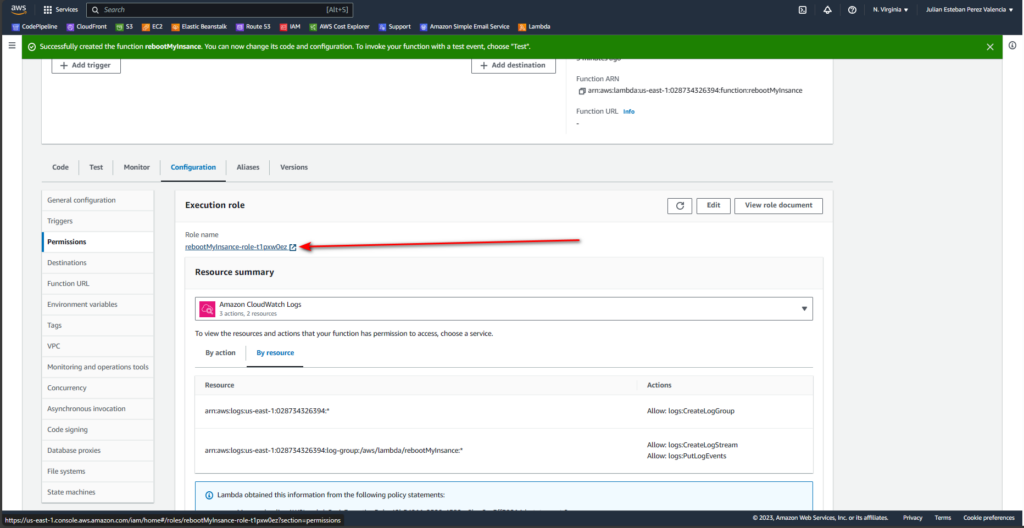
IAM Roles
We are going to add a Permissions policies item in IAM Roles. Click on Add permissions > Attach policies. In the next screen type “Lightsail” in the Search input and check Lightsail_policy. Click on Add permissions button. Now you can see Lightsail_policy in the Permissions list. If you are curious about the permissions, you’ve added click on the expand button.
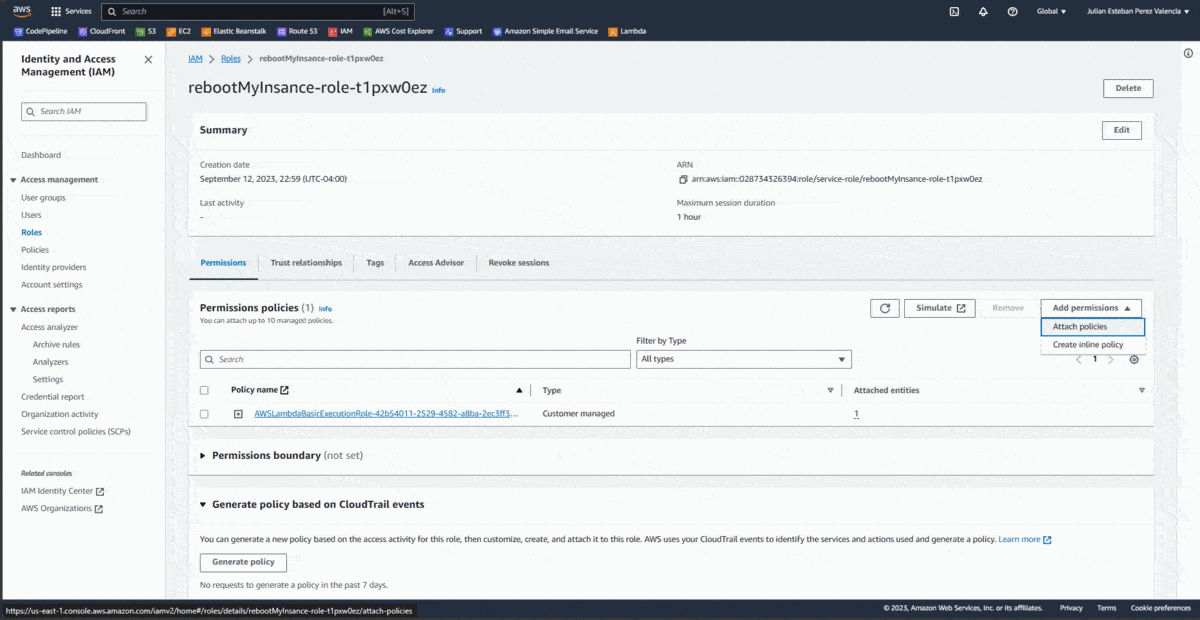
Now it’s time to deploy the code. Go to your lambda function Code and click on Deploy button.
EventBridge
Finally, add the trigger for your Lamda function. Click on + Add trigger button. Select EventBridge (CloudWatch Events). Select Create a new rule, name your rule, select Schedule expression and define the expression. Use rate() for creating a frequency expression using now time as reference or cron() for a more precise definition. Use FreeFormatter.com to create your customized cron expression. I removed the first number (seconds) from the expression because seems to be not valid fro AWS. I decided to trigger the event at 6am everyday so my expression looks as follows:
cron(0 6 ? * * *)
It’s important to keep in mind that this format uses UTC time so adapt the time for your time zone.
Click on Add button and all done.
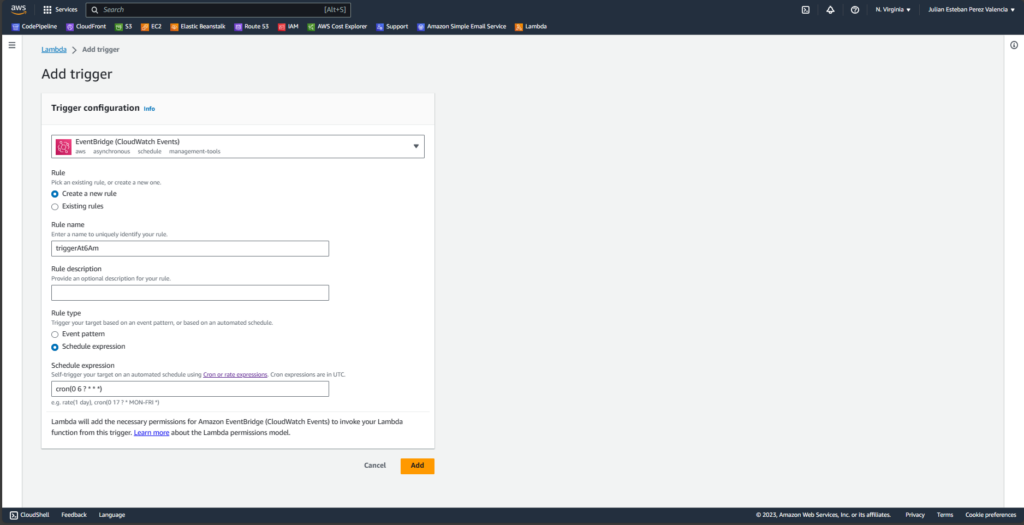
If you want to test your script, click on Test button. Lambda would print a statusCode 200. Open a new browser tab and go to your website. You should see a 503 error for a minute.
Interact with Lightsail, explore Boto3
You can explore Boto3 documentation for more actions. You can turn off an instance, get metrics, create instance snapshot, and a lot more starting from the script of this article. Hope this article could help you interacting with your Lightsail instance.